Syncing a Local Directory With a Remote Directory via FTP
Syncing a Local Directory With a Remote Directory via FTP
Overview
We can use the following Sequence Diagram to depict the process of modifying the Files within a Local Directory and a Remote Directory before syncing the two Directories via the File Transfer Protocol (FTP), a standard communication protocol used on computer networks to send files between a Client and a Server.
sequenceDiagram
loop
Machine With the FTP Client ->> Machine With the FTP Client: Modify Files
Machine With the FTP Server ->> Machine With the FTP Server: Modify Files
Machine With the FTP Server ->> Machine With the FTP Server: Start FTP Server
Machine With the FTP Client ->> Machine With the FTP Client: Start FTP Client
Machine With the FTP Client ->> Machine With the FTP Server: Connect to FTP Server
Machine With the FTP Client ->> Machine With the FTP Server: Mirror Remote Directory -> Local Directory
Machine With the FTP Server -->> Machine With the FTP Client: Update Local Directory With Changed Files in Remote Directory
Machine With the FTP Client ->> Machine With the FTP Server: Reverse Mirror Local Directory -> Remote Directory
Machine With the FTP Client -->> Machine With the FTP Server: Update Remote Directory With Changed Files in Local Directory
end
Setting Up the Machine With the FTP Client
Obviously, we need to install an FTP Client on this Machine. Personally, I recommend installing lftp
, a "sophisticated" file transfer program. Unlike a standard FTP Client, which only enables you to upload or download files, lftp
additionally enables you to maintain file synchronisation using its built-in mirror
command.
Setting Up the Machine With the FTP Server
We would also need an FTP Server on the other Machine. Although there are many existing UNIX FTP servers, such as proftpd
and vsftpd
, they are usually tricky to compile, configure, and set up and require Root Privileges to run, which is tedious, if not impossible, in many situations.
As an alternative, we write our own FTP Server using pyftpdlib
, a pure Python FTP server library written which offers a high-level interface to creating portable and efficient FTP servers. Such a solution requires us to have a Python environment running on the Machine With the FTP Server and install pyftpdlib
, which is very simple in today's world where the Python ecosystem is ubiquitous.
After setting up a Python environment and installing pyftpdlib
, we can write a script for an FTP server. Below is the script that I am using. By default, it sets up a user user
with password 12345
and listens on port 2121
of the Machine With the FTP Server's Outbound IP Address, but these settings can all be tweaked by providing command-line arguments.
1 |
|
Save this to a file, and run chmod +x
on the file to make it executable.
1 |
|
Demonstration
Last but not least, we will present a demonstration of syncing a Local Directory With a Remote Directory via FTP.
Our Local Directory is a directory named mirror_ubuntu
with two files mirror_ubuntu_1.txt
and mirror_ubuntu_2.txt
on the Machine With the FTP Client.

Our Remote Directory is a directory named mirror_ipad
with two files mirror_ipad_1.txt
and mirror_ipad_2.txt
on the Machine With the FTP Server, which is the iSH app running within an iPad.

We start the FTP Server on the Machine With the FTP Server, and start the FTP Client on the Machine With the FTP Client.
As depicted in Overview, we first Mirror Remote Directory to Local Directory, which can be accomplished by running mirror --continue --no-perms <Remote Directory> <Local Directory>
within lftp
.
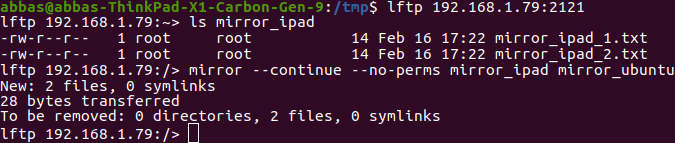
The Local Directory will now contain Files that were modified the Remote Directory.

Afterwards, we Reverse Mirror Local Directory to Remote Directory, which can be accomplished by running mirror --continue --no-perms --reverse <Local Directory> <Remote Directory>
within lftp
.

The Remote Directory will now contain Files that were modified the Local Directory.

At this point, the Local Directory has been successfully synced with the Remote Directory.
References
- https://www.geekbitzone.com/posts/lftp/lftp-mirror-remote-folders/
- https://github.com/giampaolo/pyftpdlib